Swagger API Docs: Designing, Testing and Deploying
This article will guide you through the process of deploying Swagger documentation, designing APIs, and testing them using Node.js, with code examples.
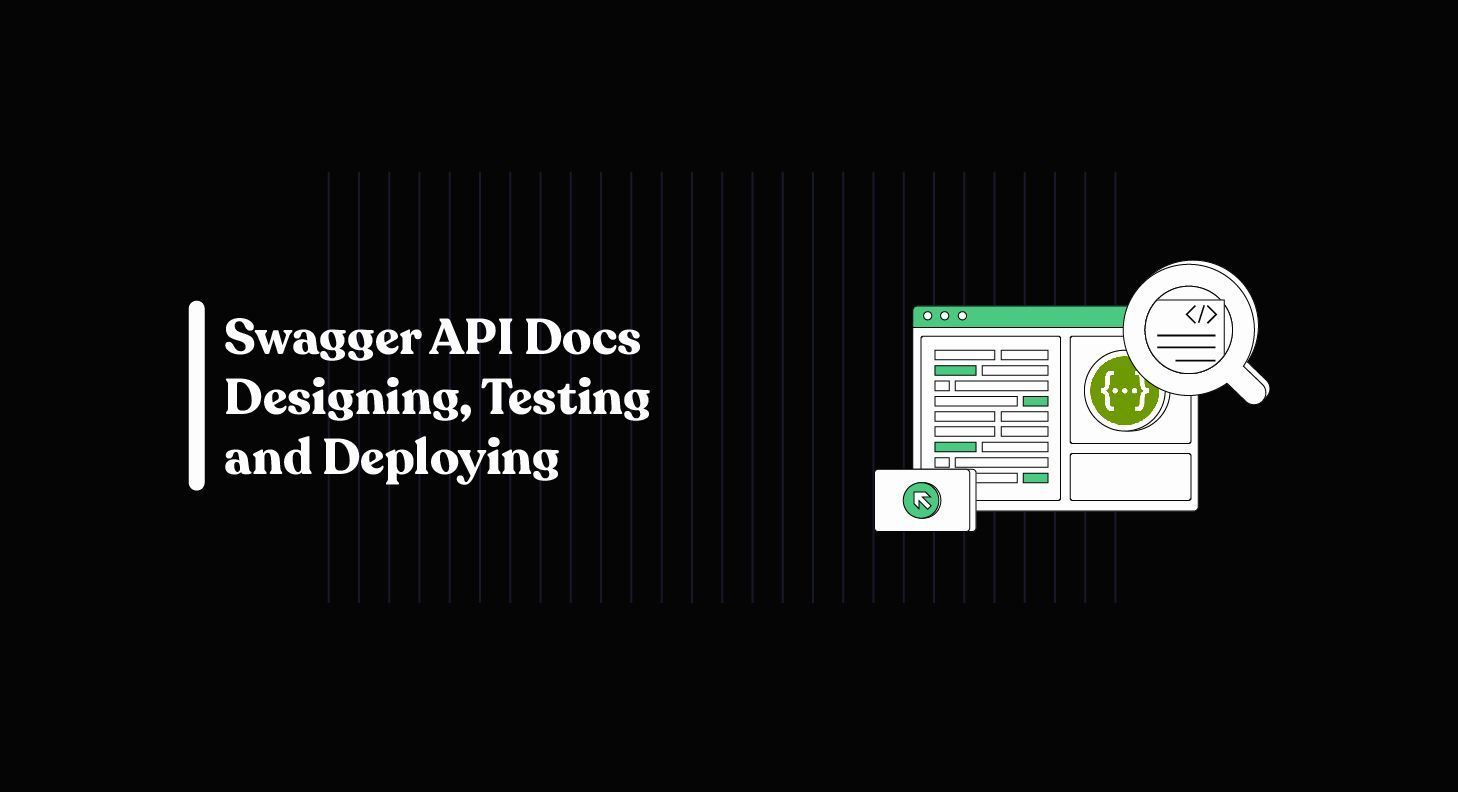
In modern software development, building robust APIs is essential for creating scalable and efficient applications. Swagger, an open-source framework, simplifies API development, documentation, and testing.
This article will guide you through the process of deploying Swagger documentation, designing APIs, and testing them using Node.js. We'll explore code examples to demonstrate each step of the process.
What is Swagger and Why Use It for API Documentation?
Swagger stands as a comprehensive toolset tailored for developing and documenting RESTful APIs. It centralizes essential API tasks, allowing developers to design, build, document, and test APIs seamlessly. Its significance lies in its multifaceted capabilities:
1. Interactivity: Swagger offers an interactive UI enabling developers to explore API endpoints and conduct real-time tests. This interactive approach simplifies understanding and adoption.
2. Standardization: Utilizing the OpenAPI Specification, Swagger ensures standardization. This specification, globally adopted, facilitates compatibility with a wide array of tools and ecosystems designed for OpenAPI, ensuring seamless integration.
3. Customizability: While Swagger provides a foundational template and UI, it grants developers complete control over the branding and styling of their API documentation. This level of customization ensures consistency with the product's overall design and user experience.
4. Open Source Advantage: Being open source, Swagger eliminates worries about usage fees or licensing costs. Developers can leverage its full potential without financial constraints, promoting widespread adoption and collaboration within the developer community.
5. Productivity Enhancement: Swagger significantly enhances productivity. It automates various time-consuming tasks, including documentation, testing, and SDK generation. The ability to generate server stubs and client SDKs directly from API specifications accelerates development cycles, ensuring API and documentation synchronization.
In essence, Swagger serves as a comprehensive toolkit, streamlining API development processes. By embracing Swagger, developers can focus on the core logic of their APIs, confident that the tool takes care of intricate documentation, testing, and synchronization intricacies.
How To Set Up Swagger in Node.js
First, let's set up Swagger in your Node.js project. Use the following steps:
Step 1: Install Swagger-UI-Express and Swagger-JSDoc
npm install swagger-ui-express swagger-jsdoc express --save
Step 2: Create a Swagger Definition File
Create a swaggerDefinition.js
file to define your API's basic information:
const swaggerDefinition = {
info: {
title: 'Test API title',
version: '0.0.1',
description: 'Test API description',
},
basePath: '/',
};
module.exports = swaggerDefinition;
Step 3: Integrate Swagger in Express App
const express = require('express');
const swaggerUi = require('swagger-ui-express');
const swaggerJSDoc = require('swagger-jsdoc');
const swaggerDefinition = require('./swaggerDefinition');
const app = express();
const options = {
swaggerDefinition,
apis: ['./routes/*.js'], // Path to the API routes
};
const swaggerSpec = swaggerJSDoc(options);
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerSpec));
Now, you can access your Swagger documentation at http://your-api-base-url/api-docs
.
Designing APIs with Swagger
Swagger provides a clean and intuitive way to design APIs. You can specify routes, request parameters, and response structures using Swagger annotations.
/**
* @swagger
* /api/route:
* get:
* description: Get data from the server
* produces:
* - application/json
* responses:
* 200:
* description: Successful operation
* schema:
* $ref: '#/definitions/ResponseModel'
*/
app.get('/api/route', (req, res) => {
// Handle the request and send a response
});
/**
* @swagger
* definitions:
* ResponseModel:
* properties:
* key:
* type: string
* value:
* type: string
*/
In this example, the /api/route
endpoint is defined with Swagger annotations. You can define request parameters, response models, and error codes similarly.
Testing APIs with Swagger
Swagger simplifies API testing by providing an interactive UI to send requests and view responses.
Interactive API Testing:
- Access your Swagger documentation at
http://your-api-base-url/api-docs
. - Navigate to the desired endpoint and click "Try it out" to interactively test the API.
- Input the required parameters and click "Execute" to send the request.
Automated Testing with Swagger-UI and Jest:
- Install necessary packages:
npm install swagger-ui-express jest supertest --save-dev
- Write a Jest test script to automate API testing:
const request = require('supertest');
const app = require('../app'); // Import your Express app
describe('Test API Endpoints', () => {
it('should return data from /api/route', async () => {
const response = await request(app).get('/api/route');
expect(response.statusCode).toEqual(200);
expect(response.body).toHaveProperty('key');
expect(response.body).toHaveProperty('value');
});
});
- Run tests using Jest:
npx jest
Conclusion
As we cover the design and testing aspects in this article, we understand that deploying APIs is a critical step that deserves attention. In our upcoming article, we’ll take a closer look at deploying Swagger-based APIs to popular platforms like Heroku and Google Cloud.